Introduction.
Setting up authentication for a web application is essential for protecting user data and controlling access. Amazon Cognito, a fully managed AWS service, simplifies this by offering user authentication, authorization, and user management capabilities for web and mobile apps. It supports user sign-up and sign-in, as well as integration with social identity providers like Google, Facebook, and Apple, or SAML-based identity providers for enterprise use. To begin, the first step is to create a Cognito User Pool. This acts as a user directory that securely handles user registration and login. Within the AWS Management Console, create a new user pool and configure it based on your app’s requirements, such as allowing users to sign up with an email or username. You can set password strength policies, enable Multi-Factor Authentication (MFA), and select required user attributes like name or phone number.
After creating the user pool, the next step is to create an App Client, which allows your web application to interact with the user pool. For front-end web applications, it’s important to disable the App Client secret, since secrets cannot be securely stored in the browser. Once the App Client is created, you can optionally configure a domain name for Cognito’s Hosted UI, which provides prebuilt, customizable sign-in and sign-up pages. OAuth 2.0 settings, such as the allowed callback and logout URLs, need to be defined to ensure proper redirection during authentication.
For integrating Cognito with your app, one popular method is using AWS Amplify, which provides a straightforward JavaScript library to handle authentication flows. After installing Amplify via npm, you configure it with your region, User Pool ID, App Client ID, and domain settings. With that done, your app can now allow users to register, sign in, and sign out using simple function calls. Additionally, Amplify provides helper methods to check the authentication state and retrieve the current user session.
Testing the authentication flow involves running your app, triggering a sign-up or sign-in process, and verifying user credentials against your Cognito User Pool. With minimal configuration and powerful features, Cognito allows developers to add secure, scalable authentication in minutes, while offloading complex identity management to AWS. Whether you’re building a personal project, a SaaS product, or an enterprise portal, Cognito gives you the flexibility to authenticate users your way.
Create a Cognito User Pool
- Go to the Amazon Cognito Console.
- Choose Create user pool.
- Choose User Pool (not Identity Pool).
- Choose Step-by-step creation.
- Configure:
- User sign-up: Enable email/username, allow self-sign-up.
- Password policy: Set your desired password complexity.
- MFA: Optional (disable or enable as needed).
- Attributes: Select email, preferred username, etc.
- Review and create the pool.
After Creation:
- Note the Pool ID and Pool ARN.
- Go to App Integration → App clients.
- Create an App Client (uncheck “Generate client secret” for web apps).
2. Configure a Domain (for Hosted UI)
- Go to App Integration → Domain name.
- Choose an AWS-hosted domain or bring your own.
- Save the changes.
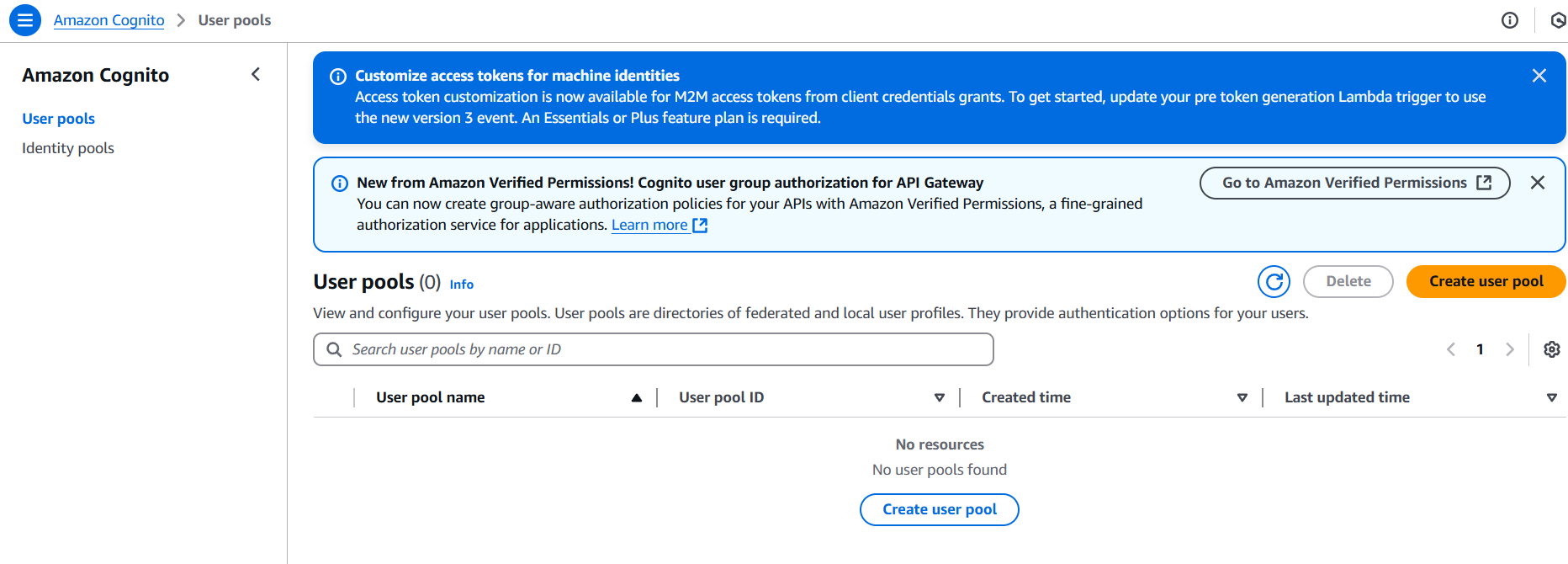
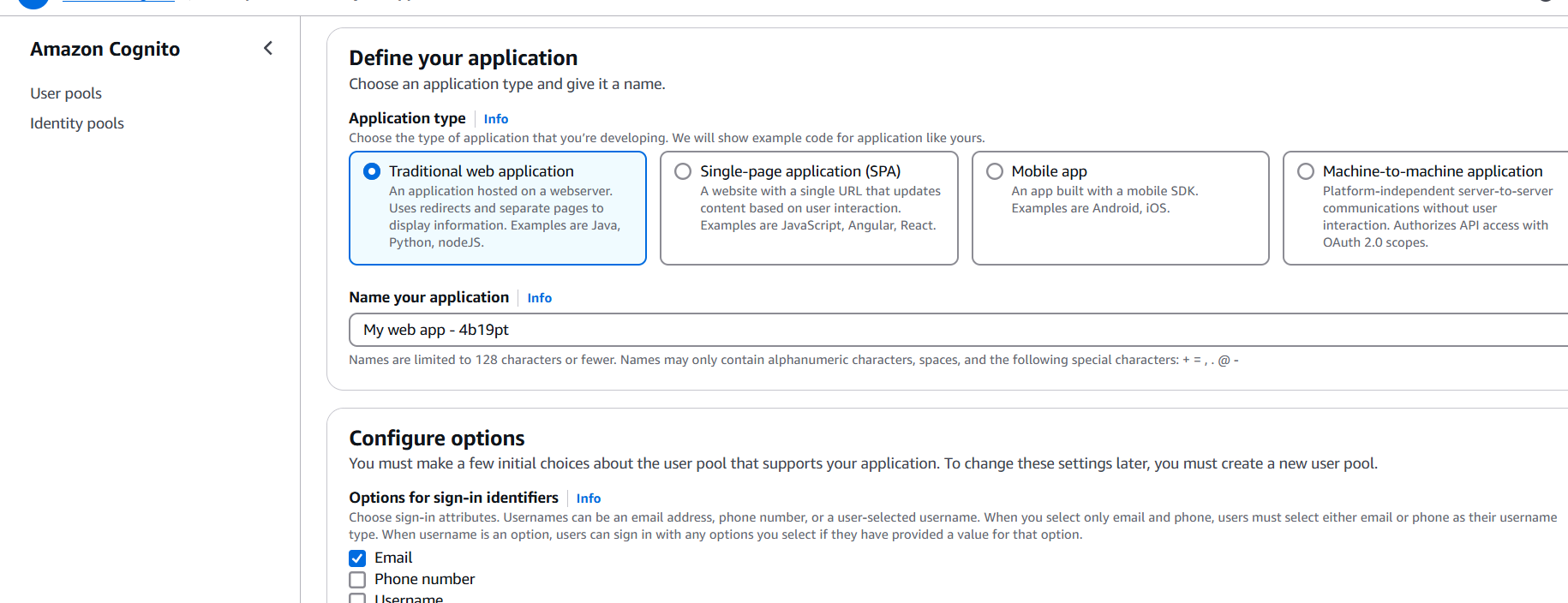
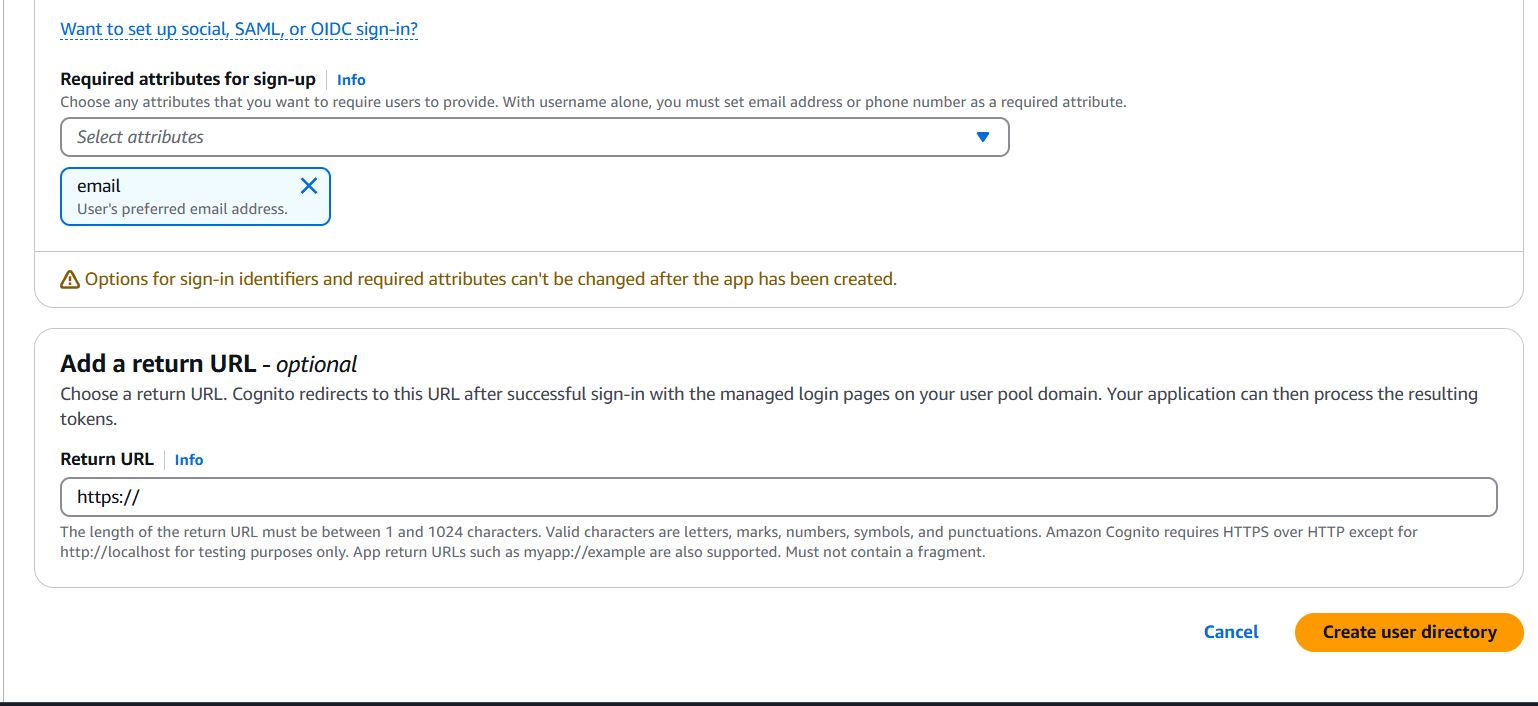
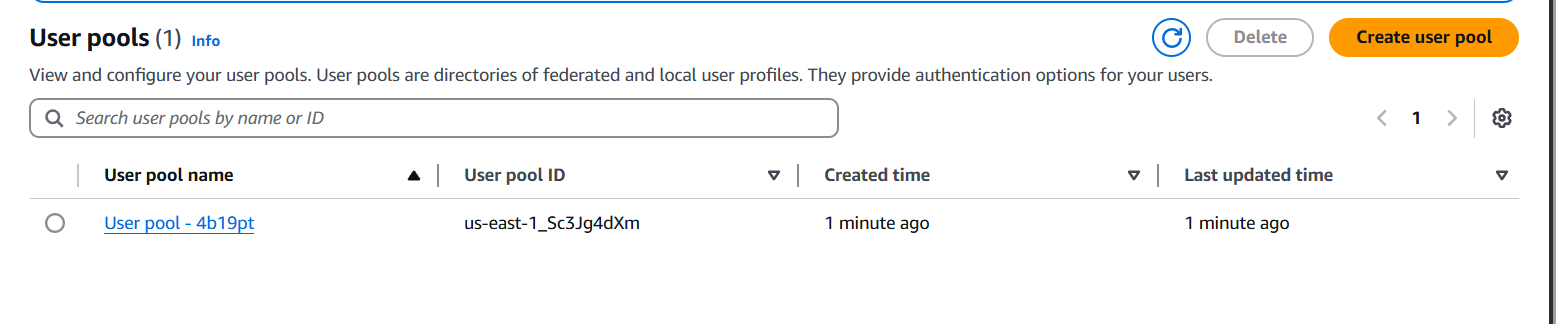
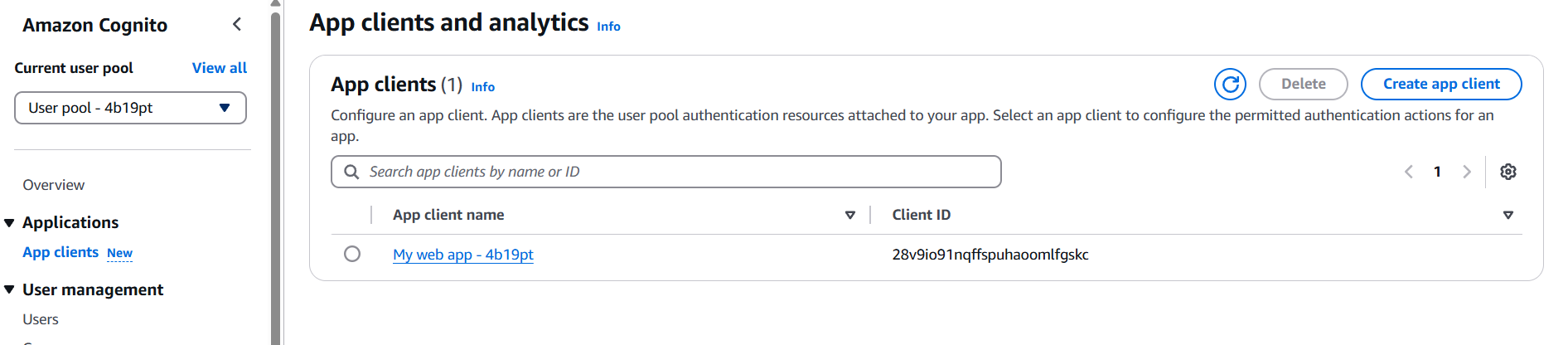
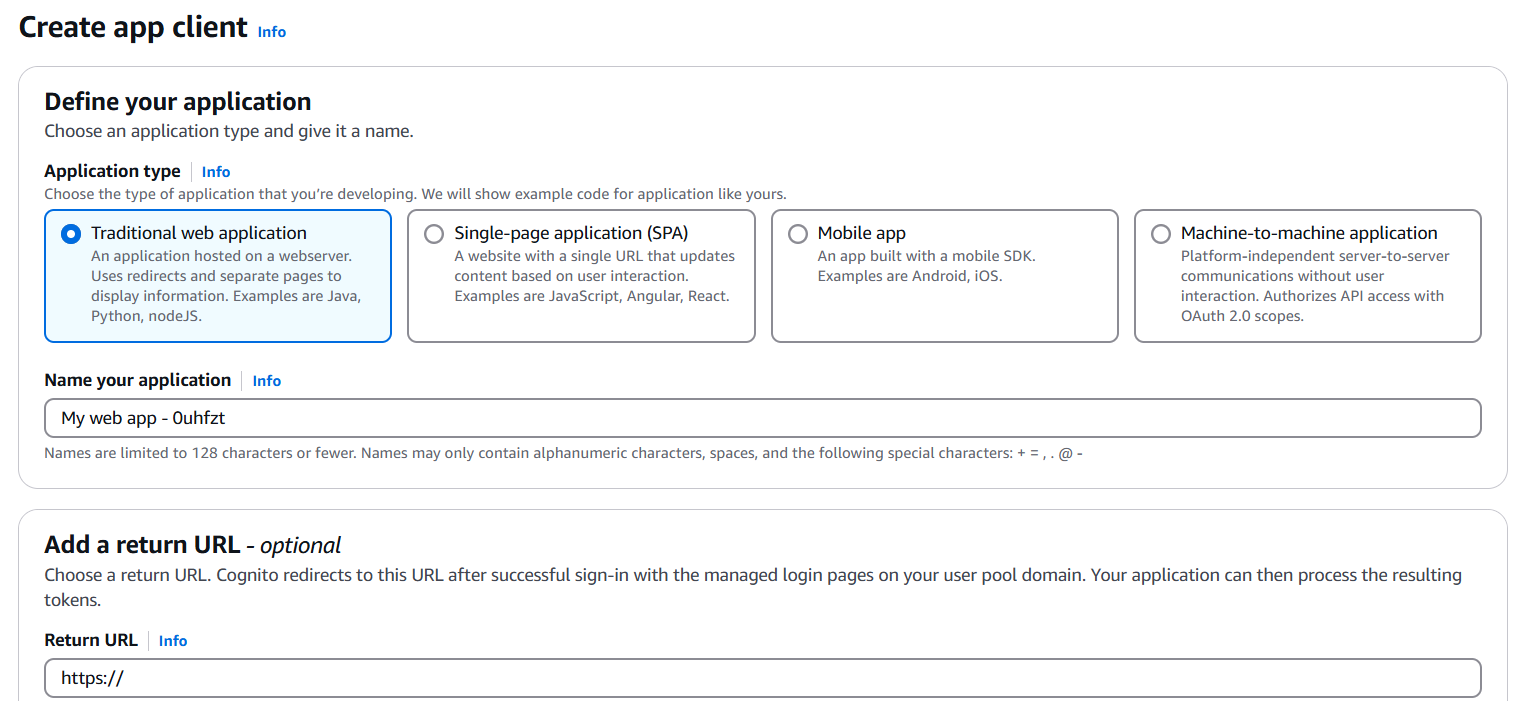
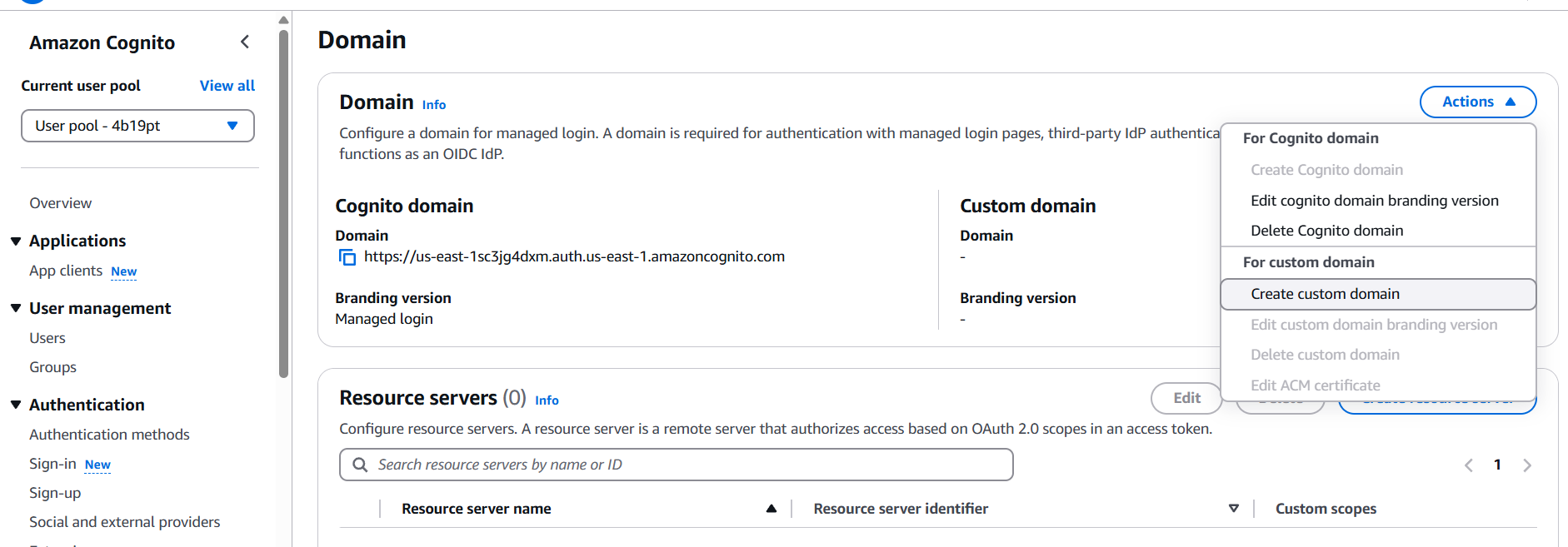
3. Set Up OAuth (Optional for Hosted UI)
Still under App Integration:
- Set up the OAuth 2.0 flow (Authorization Code Grant or Implicit grant).
- Allowed callback URLs:
http://localhost:3000/callback
(for local testing) - Allowed sign-out URLs:
http://localhost:3000/
4. Integrate Cognito in Your Web App
Use Amazon Cognito Identity SDK or AWS Amplify. Here’s a simple example using AWS Amplify.
Install AWS Amplify
npm install aws-amplify
Configure Amplify
// src/aws-exports.js (or in your code directly)
import { Amplify } from 'aws-amplify';
Amplify.configure({
Auth: {
region: 'YOUR_AWS_REGION',
userPoolId: 'YOUR_USER_POOL_ID',
userPoolWebClientId: 'YOUR_APP_CLIENT_ID',
oauth: {
domain: 'your-domain.auth.YOUR_REGION.amazoncognito.com',
scope: ['email', 'openid'],
redirectSignIn: 'http://localhost:3000/',
redirectSignOut: 'http://localhost:3000/',
responseType: 'code' // or 'token' for Implicit flow
}
}
});
Add Sign In / Sign Up Functionality
import { Auth } from 'aws-amplify';
// Sign up a new user
Auth.signUp({
username: 'email@example.com',
password: 'Password123!',
attributes: { email: 'email@example.com' }
});
// Sign in
Auth.signIn('email@example.com', 'Password123!');
// Sign out
Auth.signOut();
Test the Flow
- Run your web app.
- Go to your hosted UI domain or initiate sign-in via
Auth.federatedSignIn()
. - Register or sign in.
- Use
Auth.currentAuthenticatedUser()
to check logged-in status.
Conclusion.
In conclusion, Amazon Cognito provides a powerful and flexible solution for adding authentication to web applications with minimal overhead. By creating a User Pool, configuring an App Client, and integrating with tools like AWS Amplify, developers can implement secure sign-up, sign-in, and user management flows without building an authentication system from scratch. Whether you’re working on a simple frontend app or a large-scale cloud-native solution, Cognito scales with your needs while handling the complexities of identity and access control. With its support for industry standards, built-in security features, and seamless AWS integration, Cognito is a smart choice for modern application authentication.
Add a Comment