Introduction.
When building modern web applications, user authentication is a crucial component for managing user identities and ensuring secure access. AWS Cognito provides a powerful solution for adding authentication, authorization, and user management to your web app without the need to handle the complexities of server-side infrastructure. With AWS Cognito, you can easily set up user pools, federate identities, and integrate multi-factor authentication (MFA) to enhance security.
In this guide, we’ll walk you through the process of setting up authentication for a sample web application using AWS Cognito. We’ll start by creating a user pool, which acts as a directory for storing user information, and configure an app client to allow your application to communicate securely with Cognito. We’ll then integrate sign-up, sign-in, and password reset functionality into your web app. Along with user registration, you’ll also learn how to manage user sessions and store authentication tokens.
Additionally, AWS Cognito allows you to integrate with other AWS services like API Gateway and Lambda, enabling you to build scalable and secure serverless applications. You’ll also have the flexibility to implement features like social logins (Google, Facebook, etc.) through federated identity pools.
By the end of this guide, you’ll have a fully functional authentication system that can handle user sign-ups, sign-ins, and secure access for your web application. AWS Cognito provides an easy-to-use solution that saves time and reduces the complexity of implementing secure authentication, helping developers focus more on building great features rather than managing user data and security. Whether you’re building a small project or scaling up to a large enterprise application, Cognito can support your authentication needs with minimal setup.
Prerequisites:
- An AWS account.
- Basic knowledge of web development (HTML, JavaScript, etc.).
- AWS CLI installed and configured (optional, for easier resource management).
- A sample web application (could be a simple HTML/JavaScript frontend).
Steps to Set Up Authentication Using AWS Cognito:
1. Create a Cognito User Pool
A User Pool is a user directory that allows you to manage sign-up and sign-in services.
- Log in to the AWS Management Console.
- Navigate to Amazon Cognito.
- Click Manage User Pools and then Create a pool.
- Enter a name for the pool (e.g.,
SampleUserPool
). - Configure pool settings:
- Sign-in options: Choose how users will sign in (email, username, or phone number).
- Password policy: Define the password requirements (optional).
- MFA (Multi-Factor Authentication): You can choose to enable MFA for added security.
- Click Next step and configure App Clients (for frontend communication with Cognito).
- Create an App Client without generating a client secret (as it’s a web app).
- Enable Cognito User Pool as the authentication provider for your app.
- Save the pool configuration.
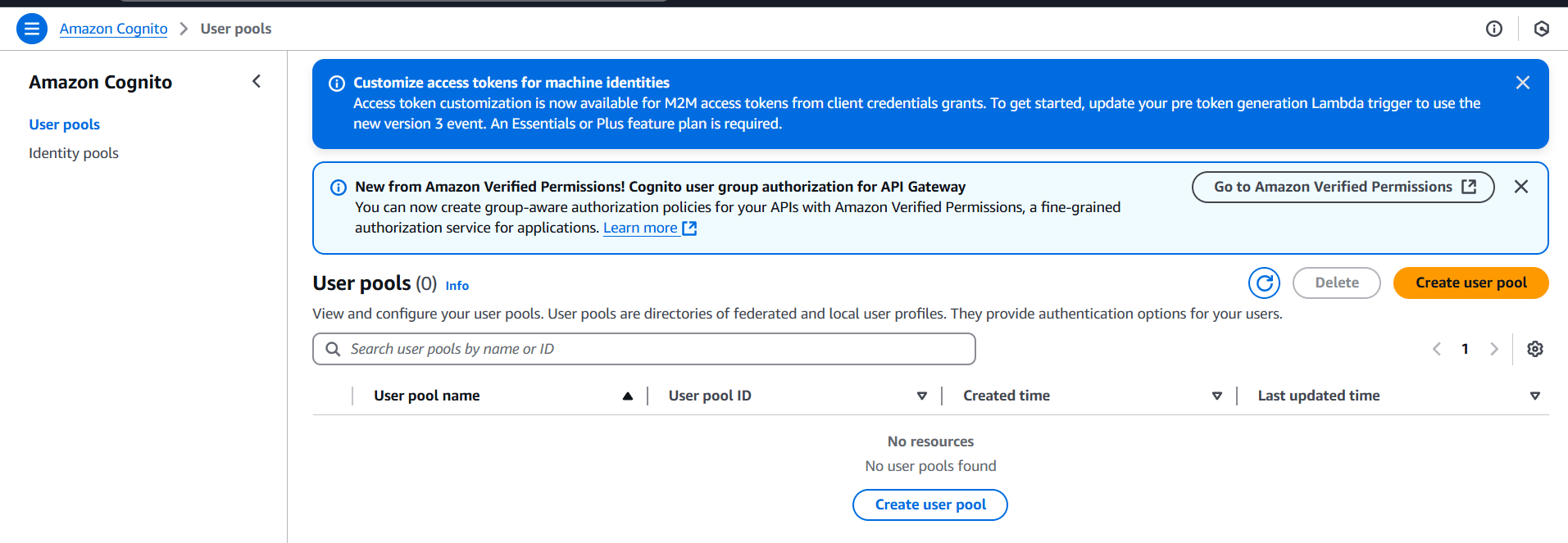
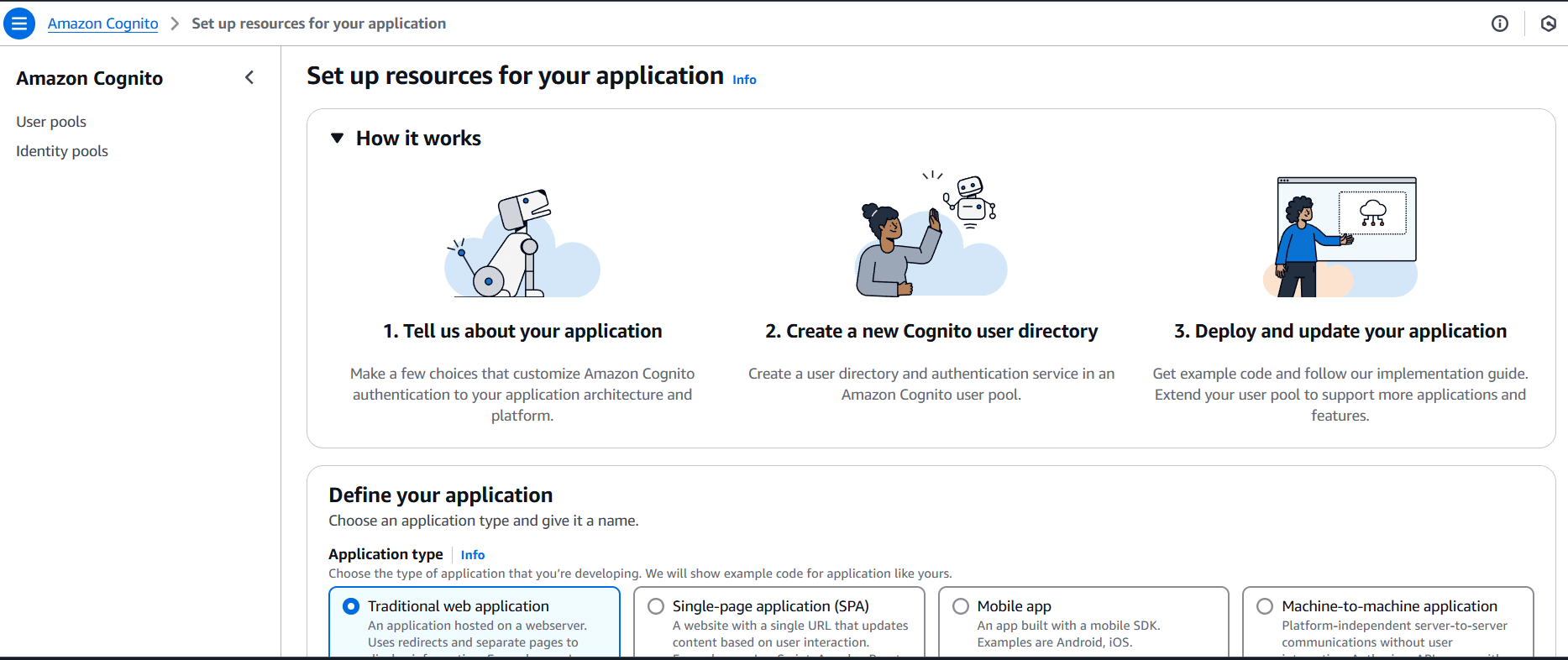
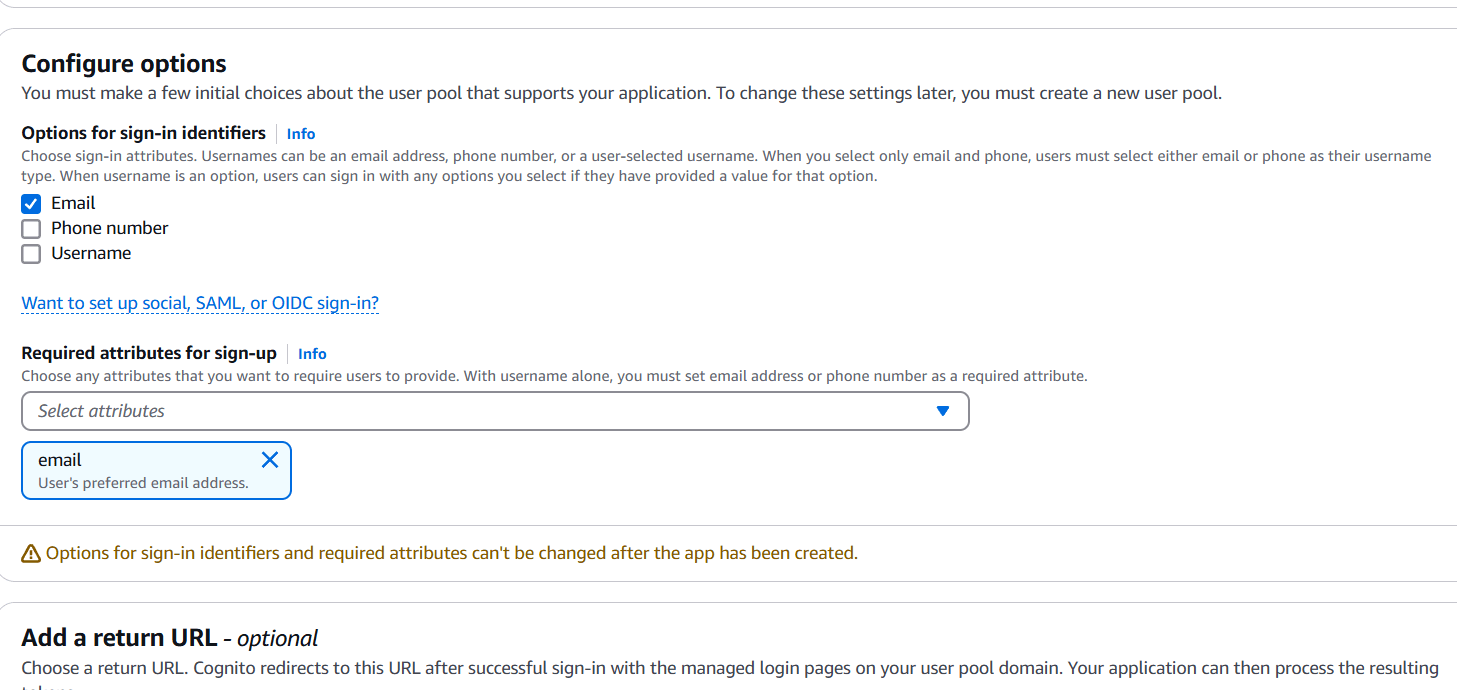
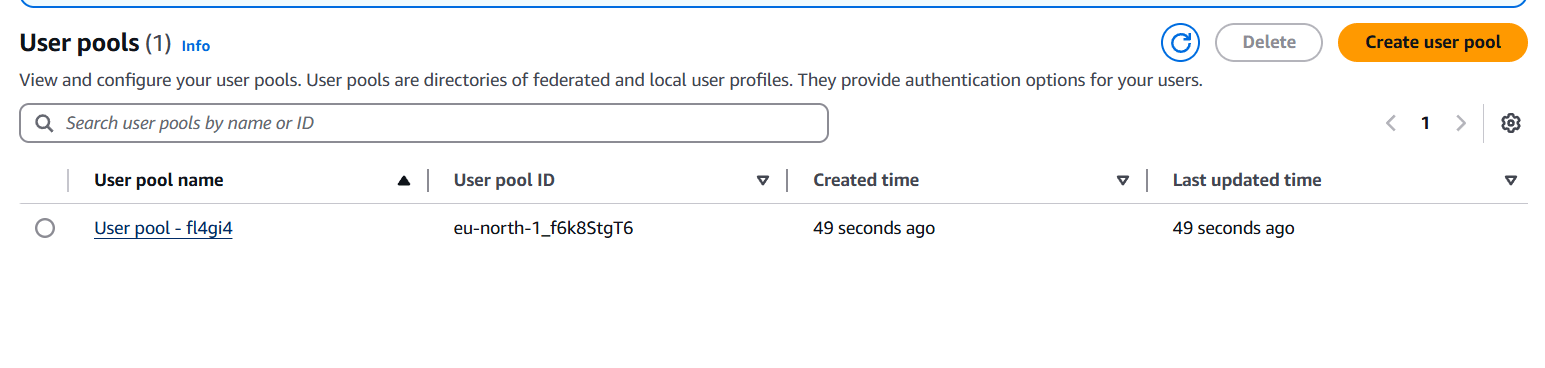
2. Set Up a Cognito Identity Pool (Optional for Federated Authentication)
If you want to access AWS services (like S3 or DynamoDB) directly from your web app, you’ll need an Identity Pool to federate the identities from the user pool into AWS services.
- From the Cognito Dashboard, click Manage Identity Pools.
- Click Create new identity pool.
- Name your identity pool (e.g.,
SampleIdentityPool
). - Enable Cognito User Pool as a provider and select the user pool you created earlier.
- Allow access to unauthenticated identities (optional, depending on your use case).
- Click Create Pool, and Cognito will create roles (IAM roles) that allow authenticated and unauthenticated users to interact with AWS services.
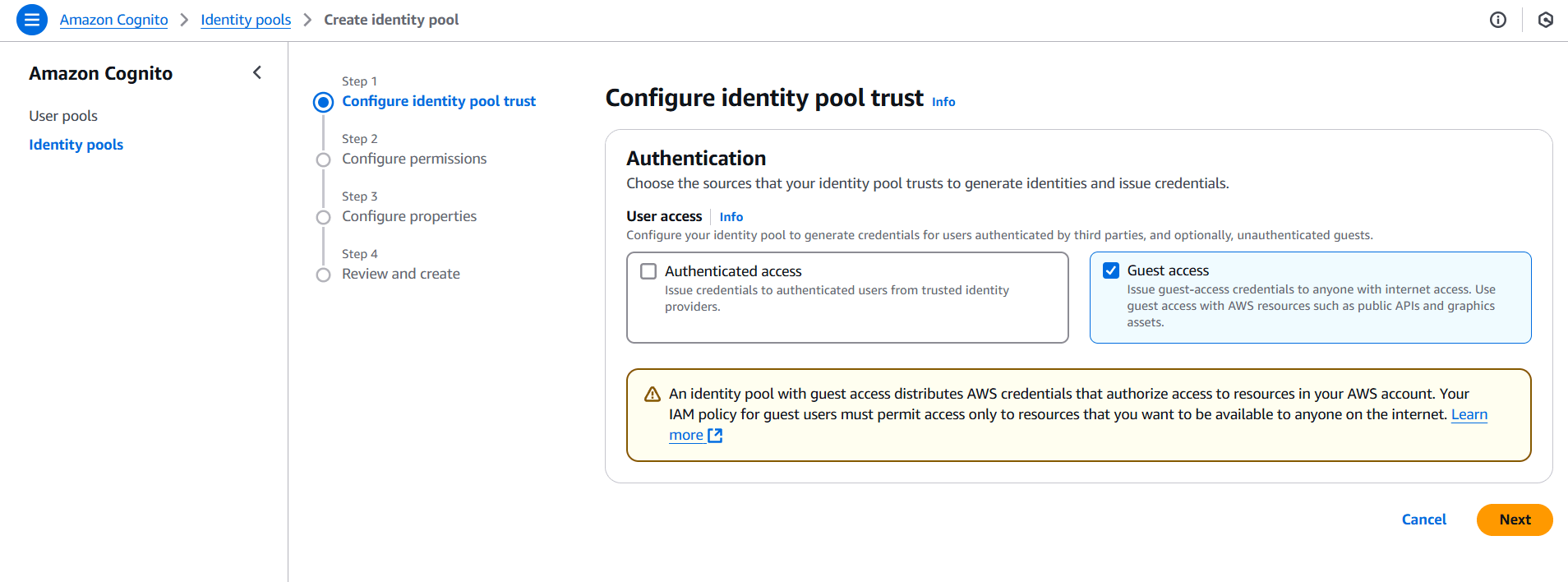
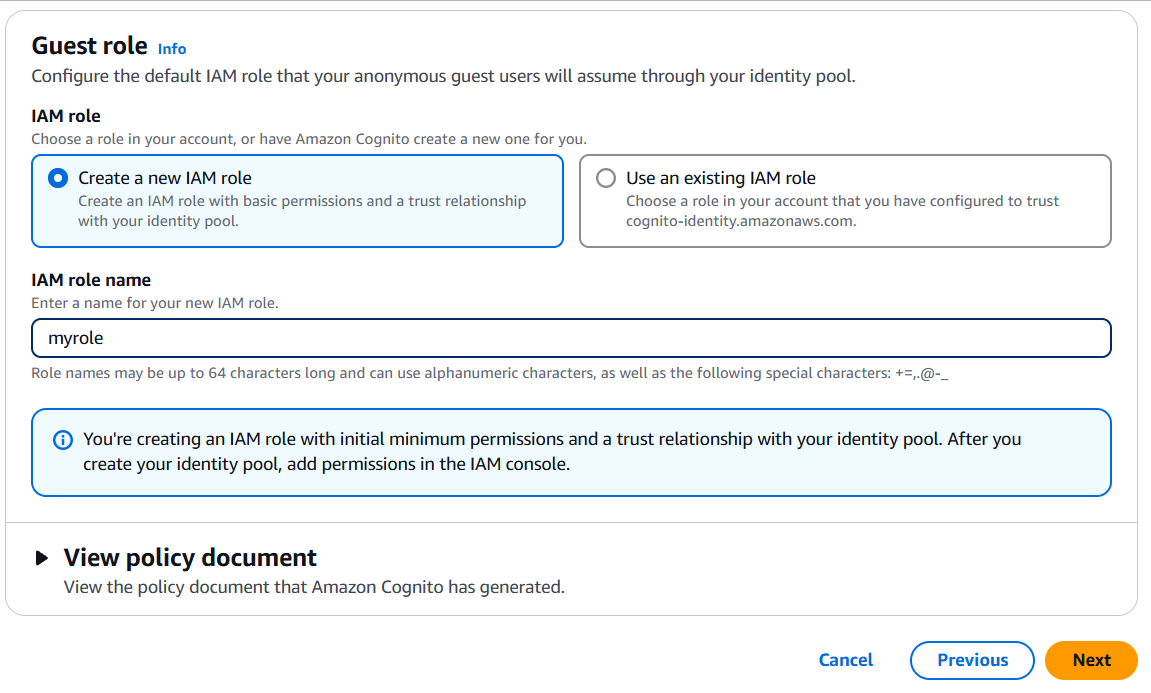
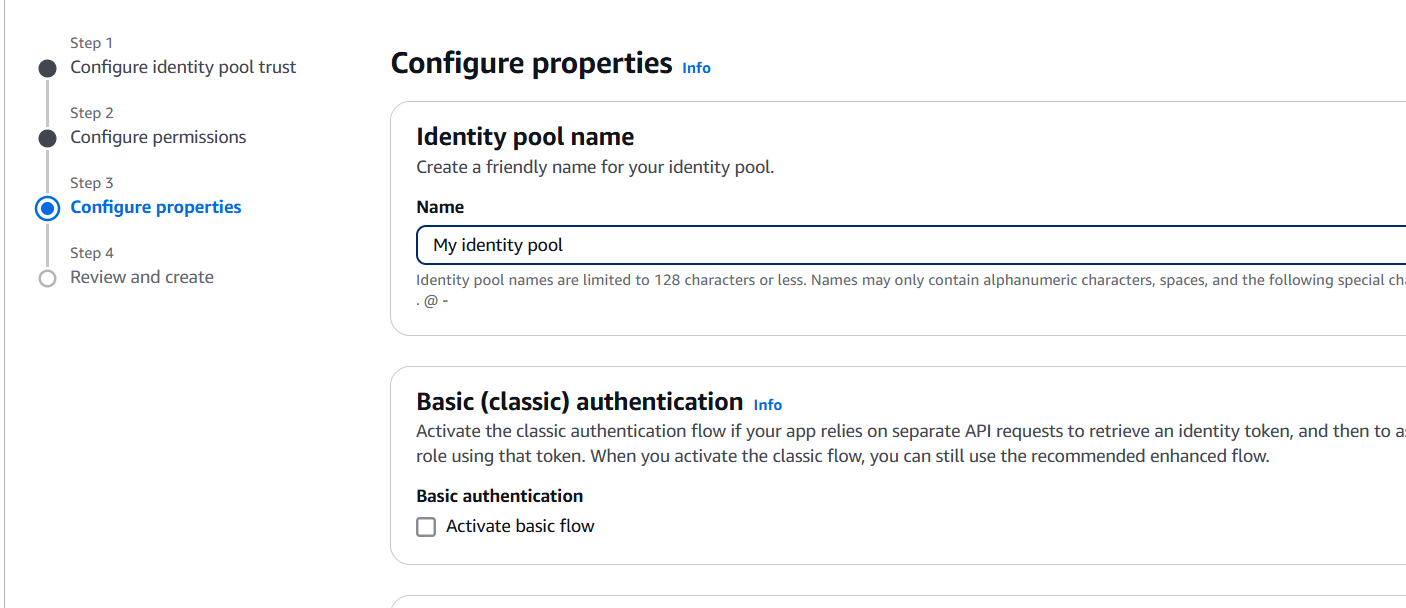
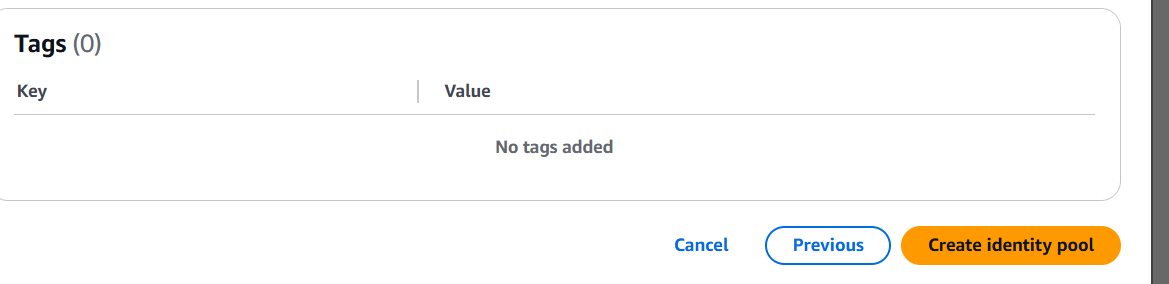
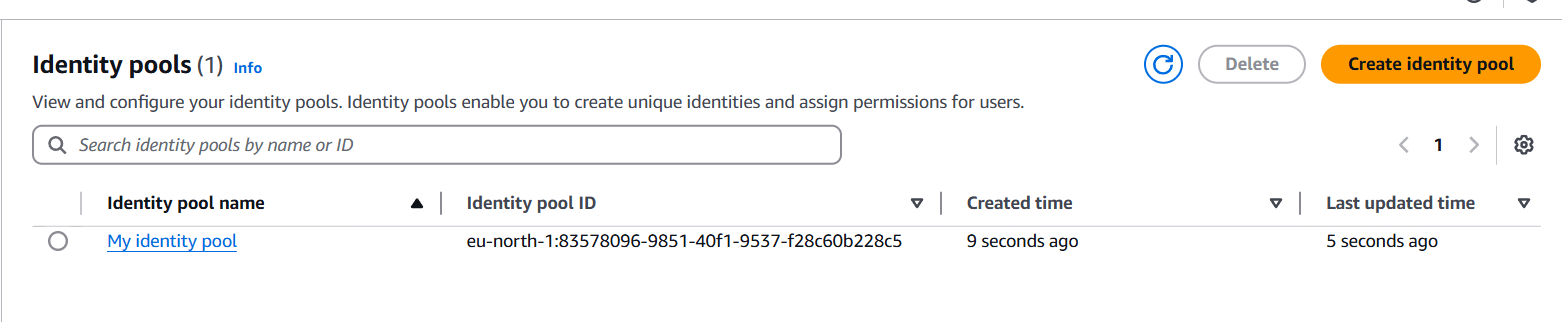
3. Configure Your Web Application
- Install the AWS SDK and Amazon Cognito Identity SDK in your web app. This can be done via a CDN or through npm.
Example: Using CDN:
<script src="https://cdn.jsdelivr.net/npm/amazon-cognito-identity-js@3.0.6/dist/amazon-cognito-identity.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/aws-sdk@2.989.0/dist/aws-sdk.min.js"></script>
4. Integrate AWS Cognito into Your Frontend (Web App)
Here’s an example of how you can integrate AWS Cognito authentication in your JavaScript frontend:
- Configure the AWS SDK and Cognito Identity Pool:
AWS.config.region = 'us-east-1'; // Your region
AWS.config.credentials = new AWS.CognitoIdentityCredentials({
IdentityPoolId: 'your-identity-pool-id', // Identity Pool ID from Cognito
});
Sign Up a New User:
const poolData = {
UserPoolId: 'your-user-pool-id', // Your User Pool ID
ClientId: 'your-app-client-id' // Your App Client ID
};
const userPool = new AmazonCognitoIdentity.CognitoUserPool(poolData);
function signUp() {
const username = document.getElementById('username').value;
const password = document.getElementById('password').value;
const email = document.getElementById('email').value;
const attributeList = [
new AmazonCognitoIdentity.CognitoUserAttribute({
Name: 'email',
Value: email
})
];
userPool.signUp(username, password, attributeList, null, function(err, result) {
if (err) {
alert(err.message || JSON.stringify(err));
return;
}
alert('Registration successful! Please verify your email.');
});
}
Sign In Existing Users:
function signIn() {
const username = document.getElementById('username').value;
const password = document.getElementById('password').value;
const authenticationData = {
Username: username,
Password: password,
};
const authenticationDetails = new AmazonCognitoIdentity.AuthenticationDetails(authenticationData);
const userData = {
Username: username,
Pool: userPool,
};
const cognitoUser = new AmazonCognitoIdentity.CognitoUser(userData);
cognitoUser.authenticateUser(authenticationDetails, {
onSuccess: function(result) {
alert('Authentication successful');
console.log(result);
},
onFailure: function(err) {
alert(err.message || JSON.stringify(err));
}
});
}
5. Handle User Authentication States
After login or signup, store the authentication tokens (JWT) locally (like in localStorage or sessionStorage) to manage the user session across different pages of your web app.
- On successful login, store the session:
cognitoUser.getSession(function(err, session) {
if (err) {
alert(err.message || JSON.stringify(err));
return;
}
console.log('Session Valid: ' + session.isValid());
localStorage.setItem('idToken', session.getIdToken().getJwtToken());
});
- On page load, you can check if the user is authenticated by verifying the presence of the ID token:
const idToken = localStorage.getItem('idToken');
if (idToken) {
console.log('User is authenticated');
} else {
console.log('User is not authenticated');
}
6. Test Your Application
- Open your application in a browser.
- Try signing up, confirming the user via email, signing in, and signing out.
- Check the console logs for responses or errors.
Conclusion.
Using AWS Cognito for authentication in a web app allows you to easily manage users, secure their data, and integrate with AWS services for seamless user experiences. With Cognito’s ability to handle user registration, login, and identity federation, you can focus on building your application while ensuring secure and scalable authentication for your users.
Add a Comment