Introduction.
Building a WordPress website using Terraform involves automating the provisioning and configuration of the infrastructure needed to run WordPress on AWS (or any other cloud provider you choose). Terraform is an Infrastructure-as-Code (IaC) tool that allows you to define your infrastructure in code and manage it consistently and efficiently.
In this guide, we’ll walk you through the process of setting up a WordPress site using Terraform to automate infrastructure provisioning on AWS.
Prerequisites:
- Terraform: Make sure Terraform is installed on your machine. You can download it from Terraform’s official website.
- VS Code: Visual Studio Code should be installed, and you should have the Terraform extension installed.
- AWS Account: An active AWS account for deploying resources.
- AWS CLI: Make sure the AWS CLI is configured to use your credentials.
TASK 1: Set up Terraform Configuration
STEP 1: Create variables.tf file.
- Enter the following command.
- And save the file.
variable "access_key" {
description = "Access key to AWS console"
}
variable "secret_key" {
description = "Secret key to AWS console"
}
variable "region" {
description = "AWS region"
}
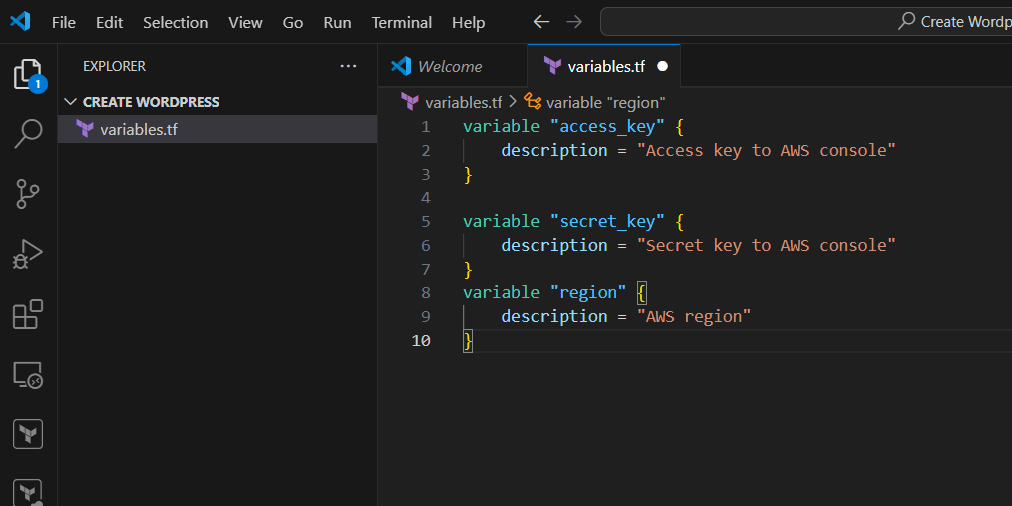
STEP 2: Next, Create terraform.tf file.
- Enter the following command and save the file.
region = "us-east-1"
access_key = "<YOUR AWS CONSOLE ACCESS ID>"
secret_key = "<YOUR AWS CONSOLE SECRET KEY>"
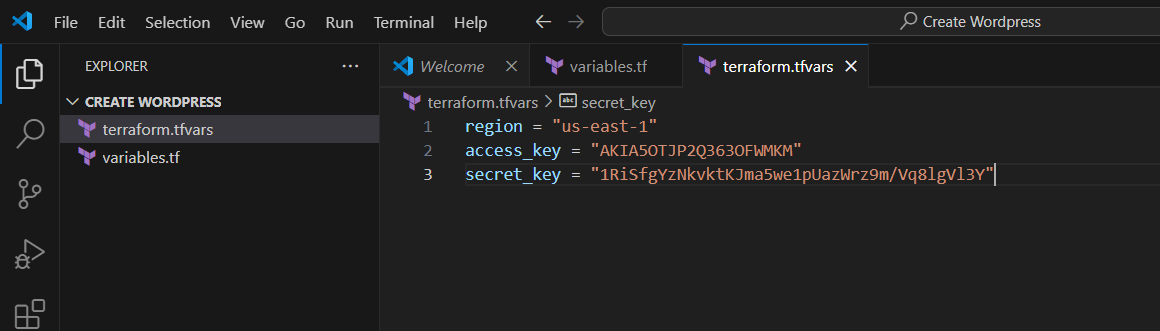
STEP 3: Create main.tf file.
provider "aws" {
region = "us-east-1" # Change region as per your preference
}
resource "aws_security_group" "wordpress_sg" {
name = "wordpress_sg"
description = "Security group for WordPress"
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 443
to_port = 443
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
resource "aws_instance" "wordpress" {
ami = "ami-0c55b159cbfafe1f0" # This should be the latest Ubuntu AMI (update as per region)
instance_type = "t2.micro"
key_name = "your-key-pair" # Ensure you have an existing key pair
security_groups = [aws_security_group.wordpress_sg.name]
tags = {
Name = "WordPress-Instance"
}
user_data = <<-EOF
#!/bin/bash
sudo apt update -y
sudo apt install apache2 -y
sudo apt install mysql-server -y
sudo apt install php libapache2-mod-php php-mysql -y
sudo systemctl start apache2
sudo systemctl enable apache2
sudo systemctl start mysql
sudo systemctl enable mysql
cd /var/www/html
sudo wget https://wordpress.org/latest.tar.gz
sudo tar -xvzf latest.tar.gz
sudo chown -R www-data:www-data /var/www/html/wordpress
EOF
}
resource "aws_eip" "wordpress_ip" {
instance = aws_instance.wordpress.id
}
output "website_url" {
value = "http://${aws_eip.wordpress_ip.public_ip}"
}
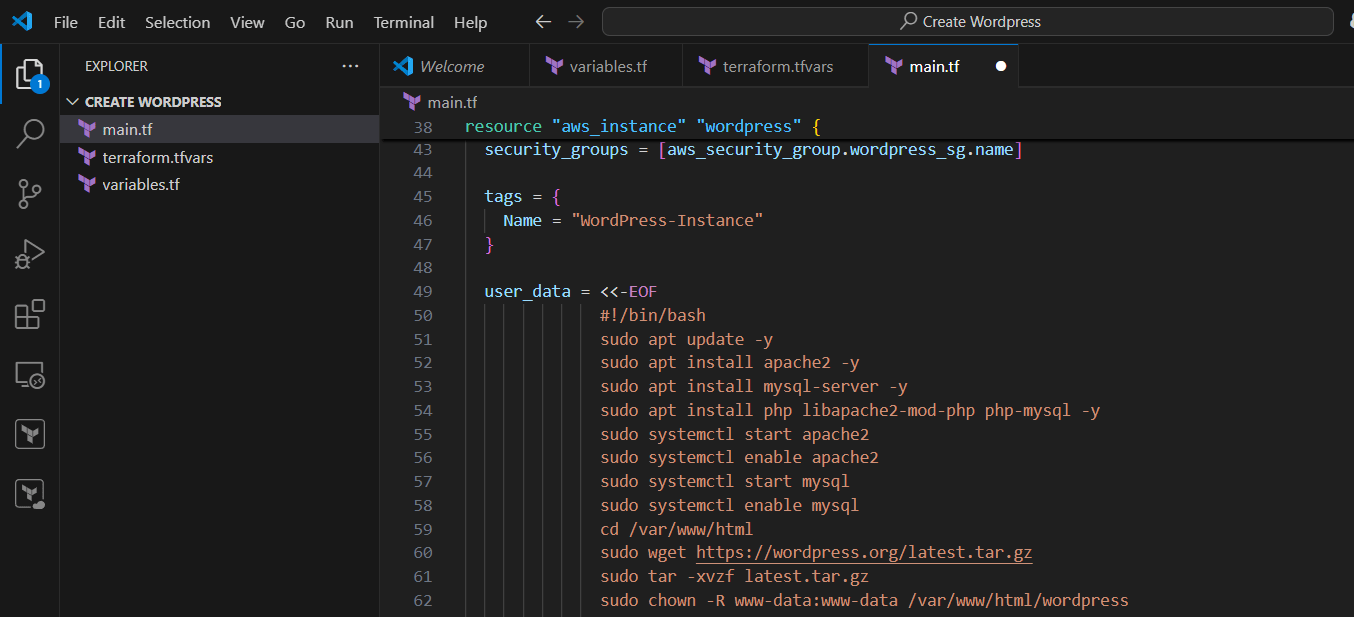
TASK 2: Apply terraform configurations.
STEP 1: Go to terminal enter terraform init command.
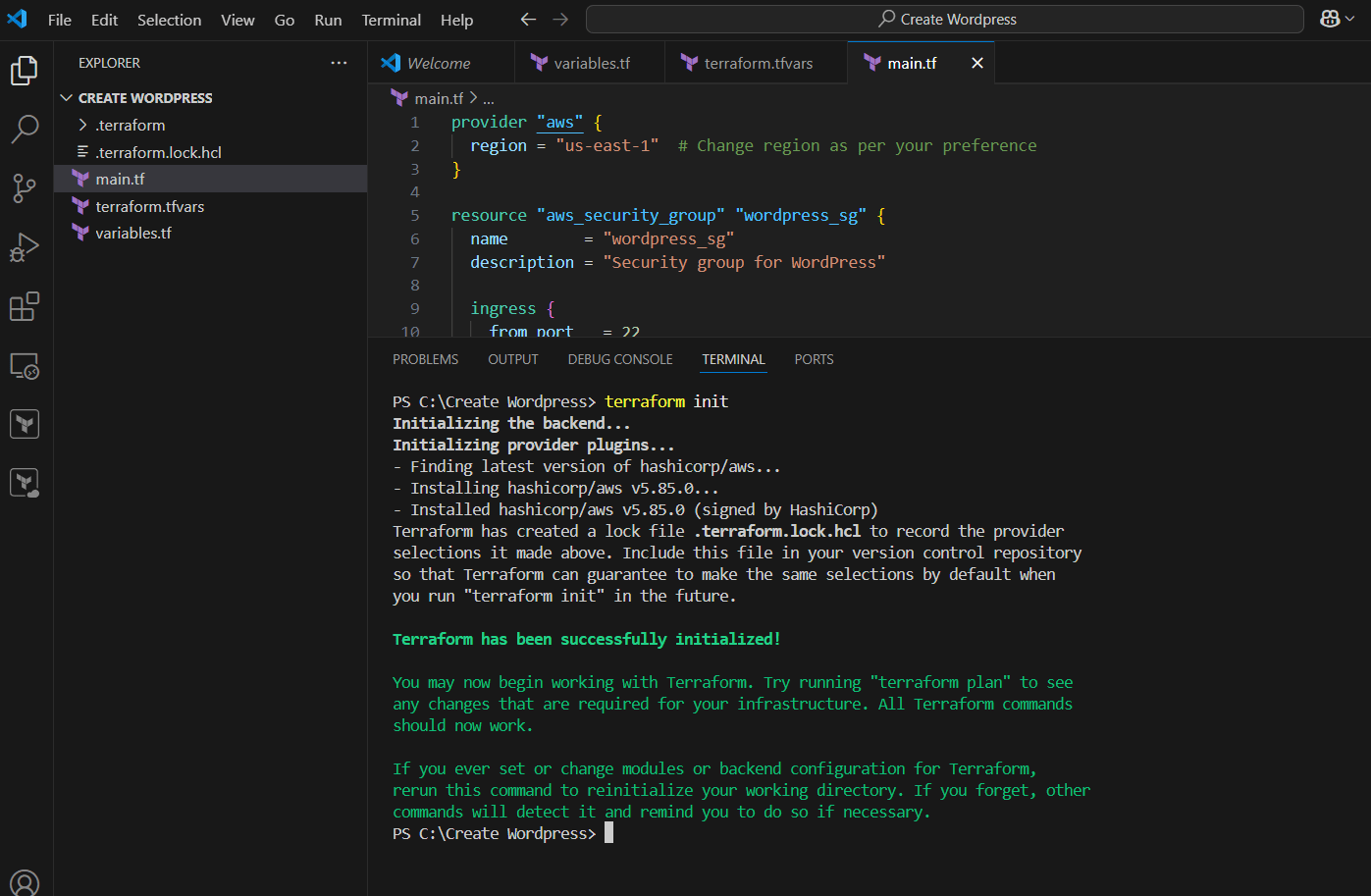
STEP 2: Next, Enter terraform plan and terraform apply command.
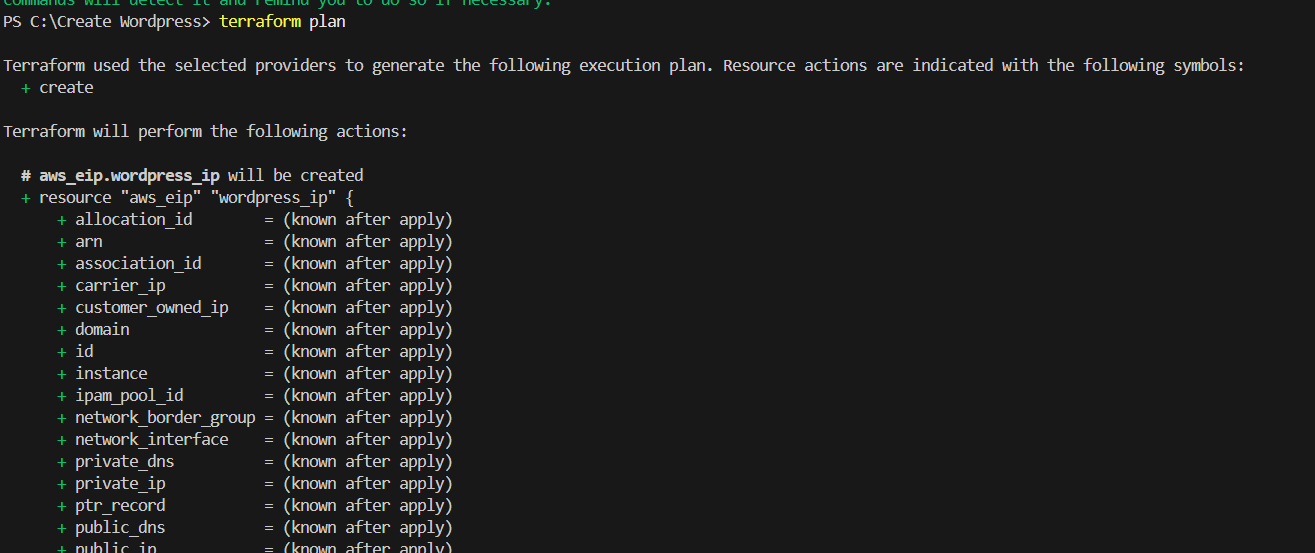
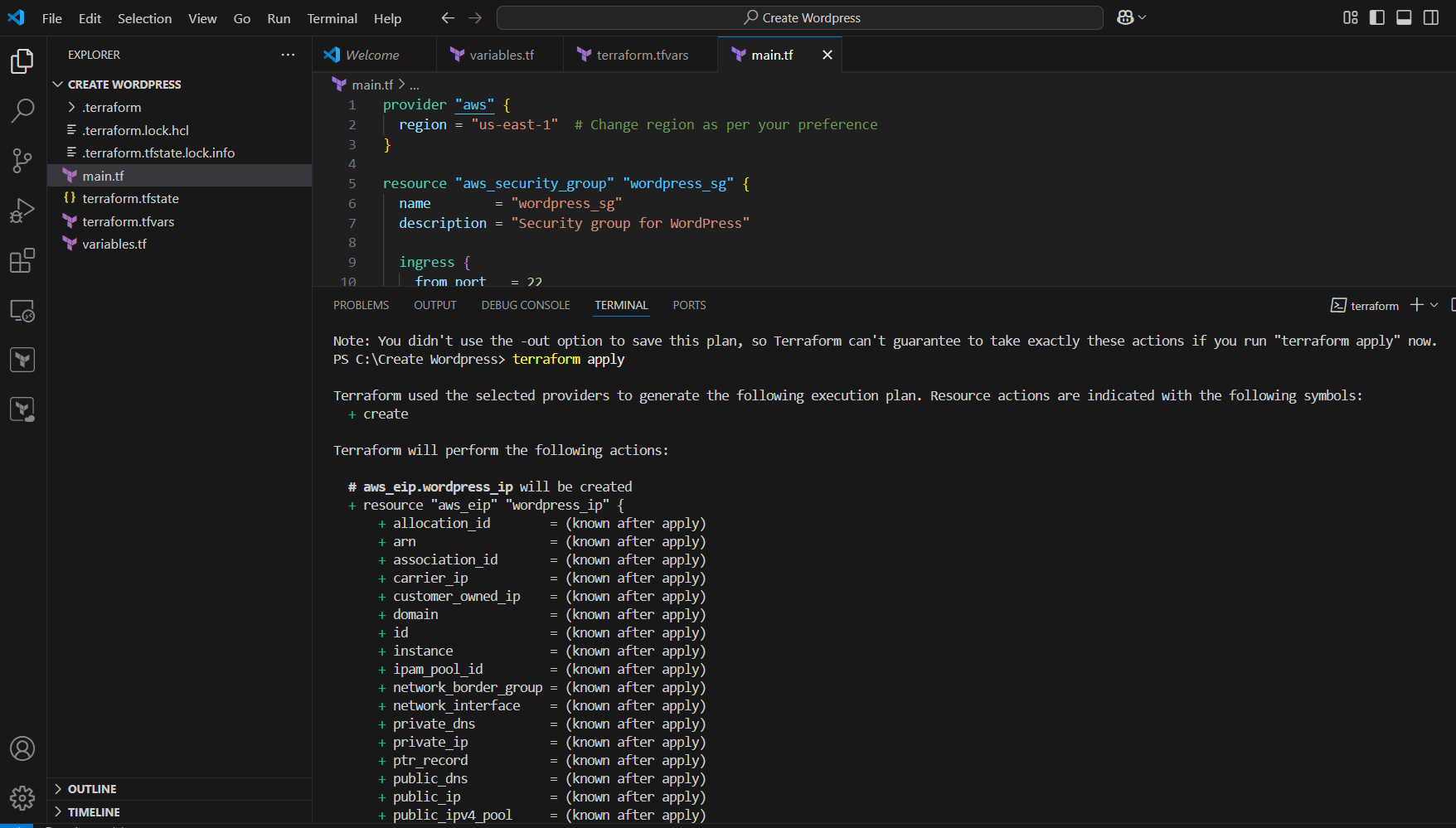
TASK 3: Open the WordPress Dashboard.
STEP 1: Go to aws console verify created the instance.
- Select the public IP and paste your browser.
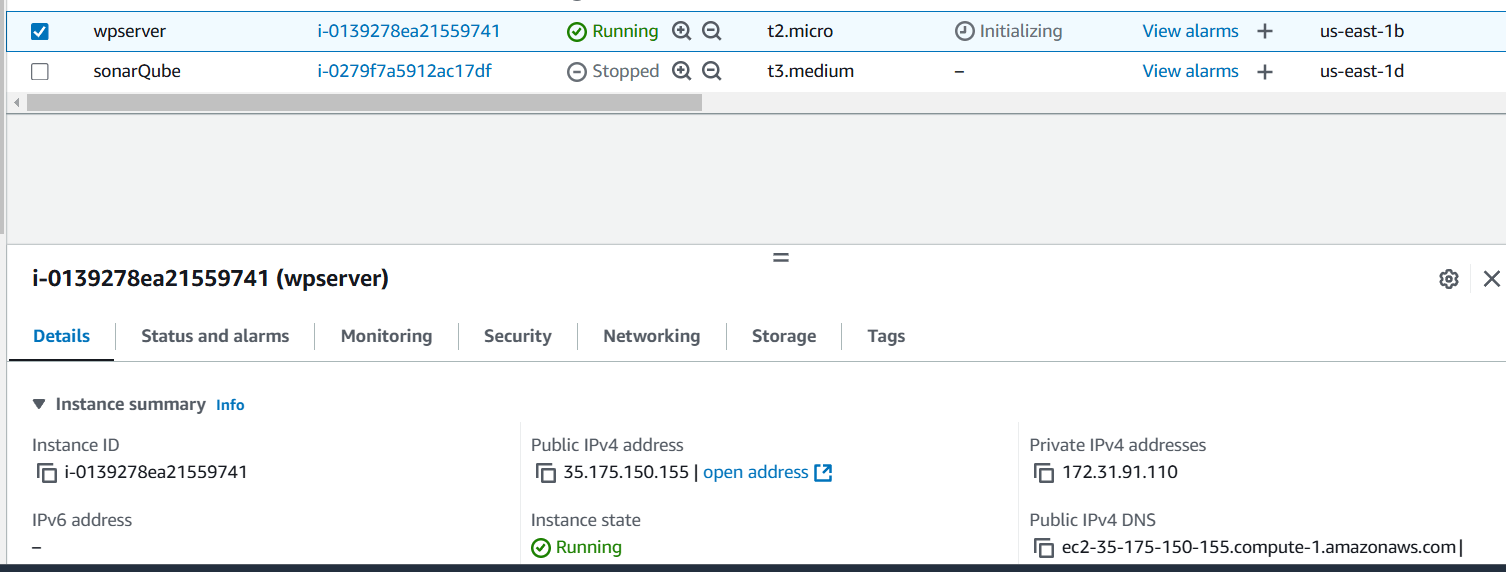
STEP 2: Select English and click continue.
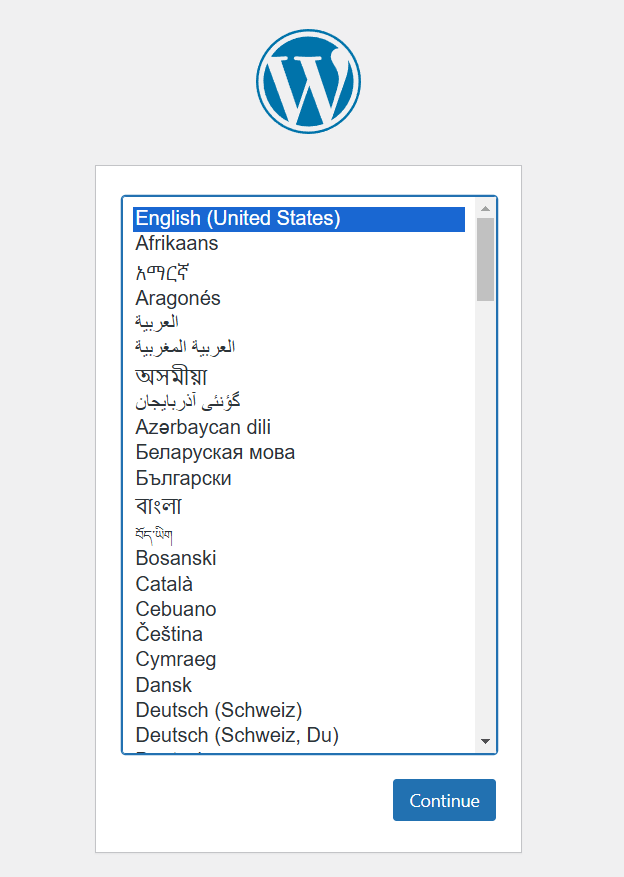
STEP 3: Enter the Title , Username , Email.
- Click on WordPress.
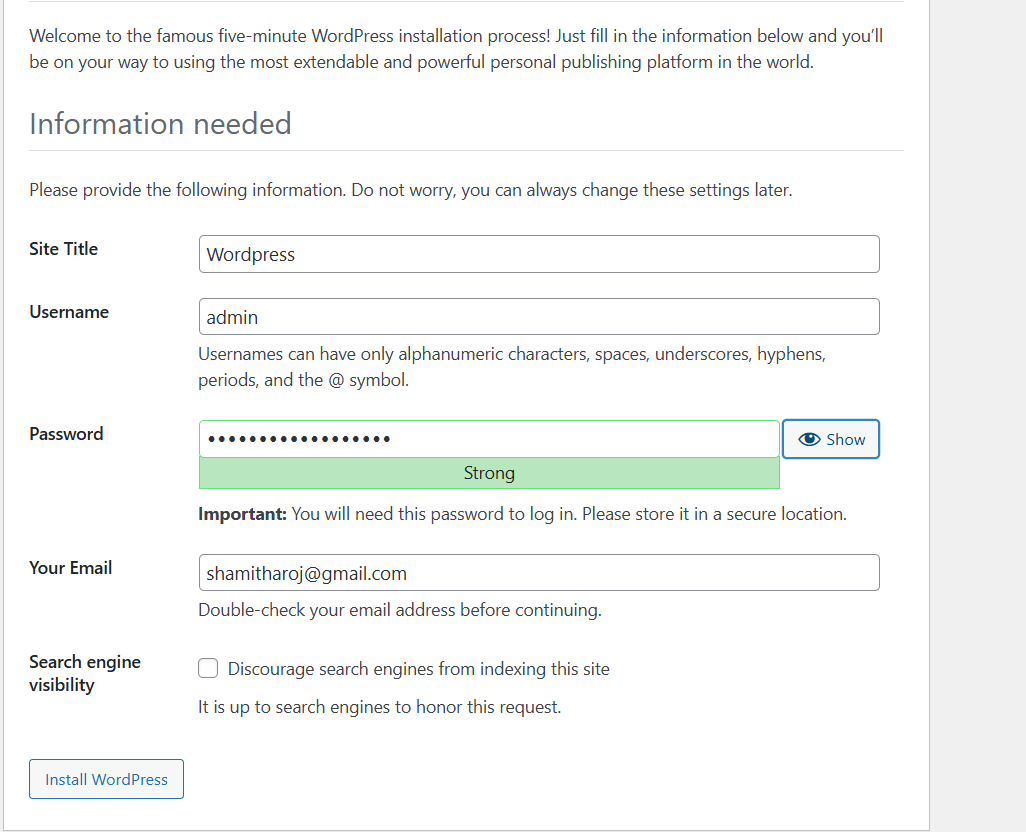
Step 4 : Click on Log In button.
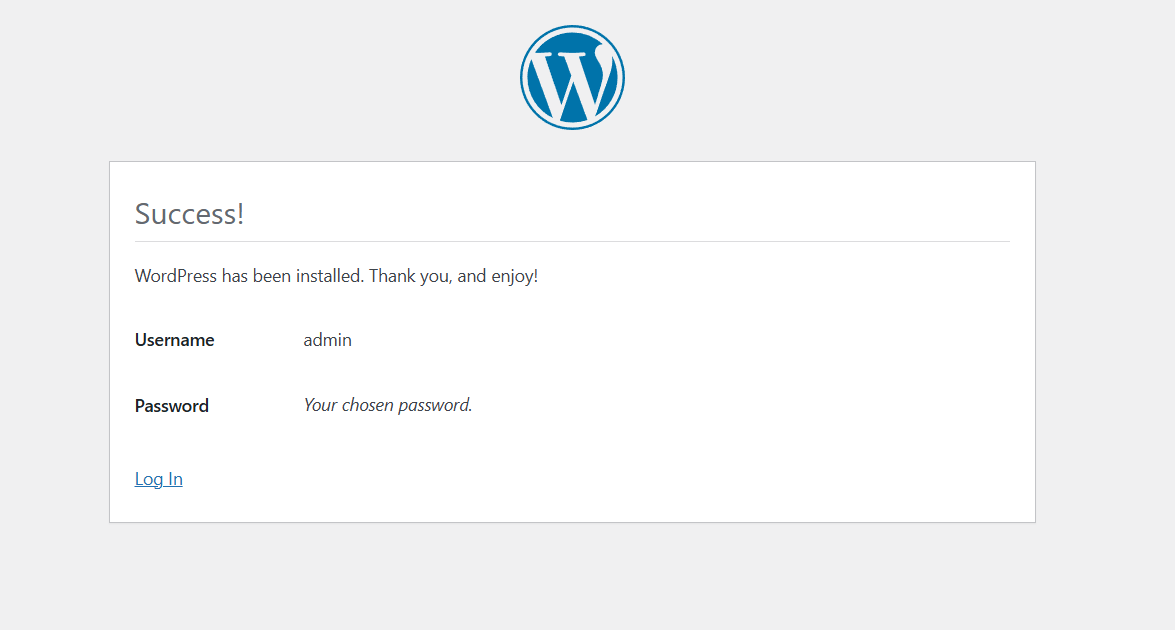
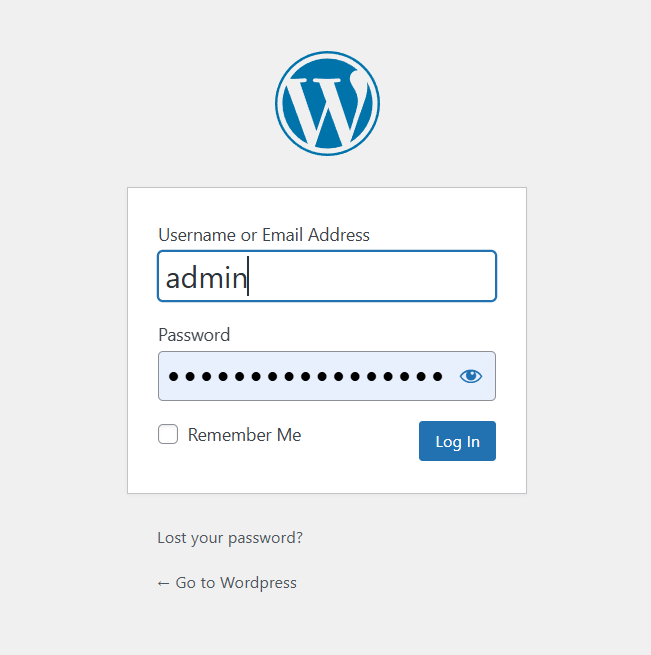
STEP 5 : Now you will see wordpress dashboard.
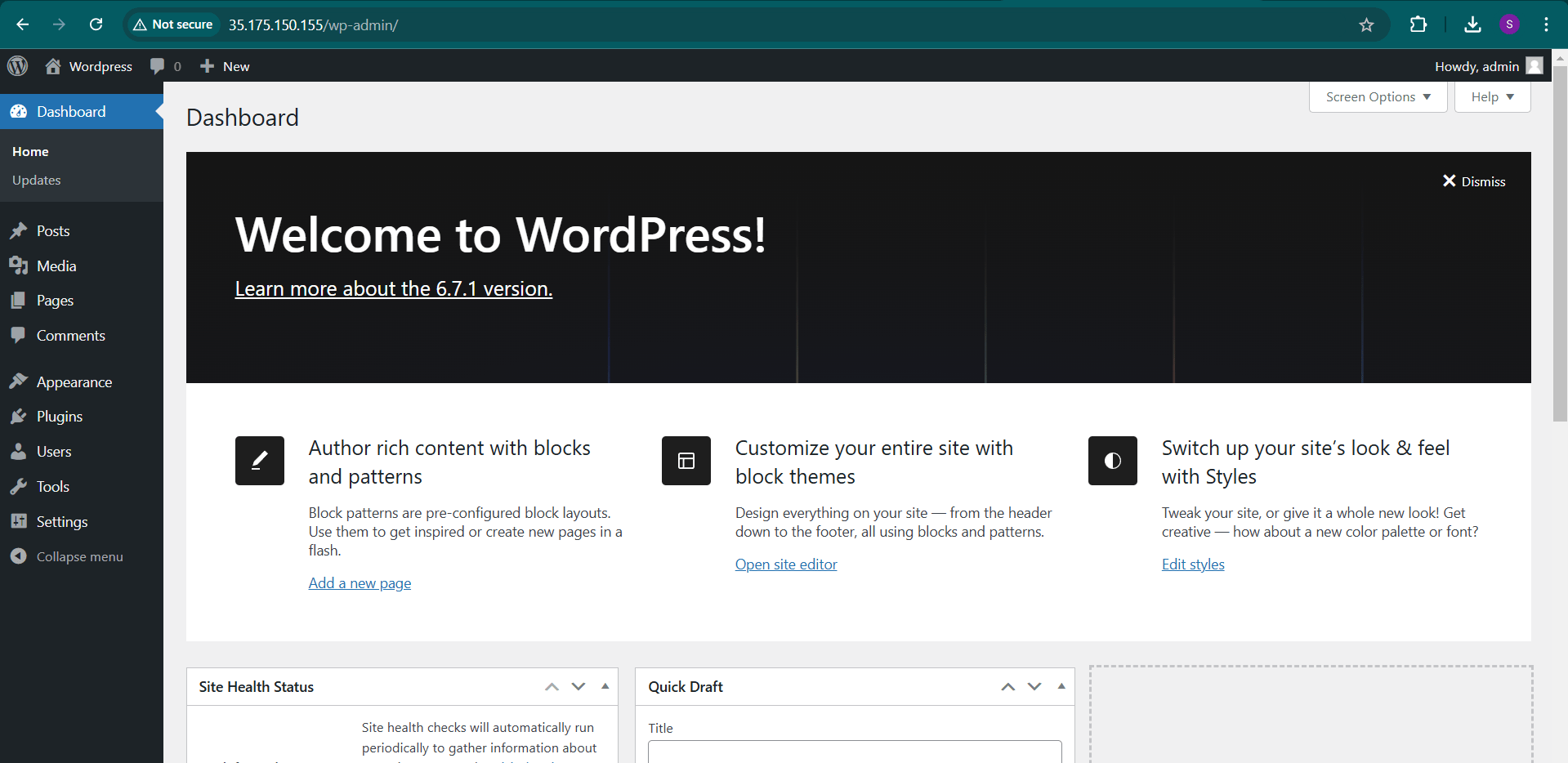
Conclusion.
Building a WordPress website using Terraform in Visual Studio Code is an excellent way to automate the infrastructure deployment process, especially when working in a cloud environment like AWS. By leveraging Terraform, you can ensure that your WordPress setup is repeatable, scalable, and easily manageable.
Add a Comment