Introduction.
In the world of modern application development, serverless architecture has become a go-to solution for building scalable, cost-effective, and event-driven systems. At the heart of AWS’s serverless ecosystem are two powerful services: API Gateway and AWS Lambda.
API Gateway acts as the front door to your backend services, allowing you to expose RESTful APIs to the internet or internal applications. AWS Lambda, on the other hand, lets you run code without provisioning or managing servers—executing logic only when needed, and charging you only for compute time used.
While it’s possible to create and connect these services manually through the AWS Console, doing so can quickly become complex, error-prone, and hard to maintain—especially as your infrastructure grows.
That’s where Terraform comes in. Terraform is an open-source Infrastructure as Code (IaC) tool that lets you define your infrastructure in simple, readable configuration files. With Terraform, you can provision, manage, and version your API Gateway, Lambda functions, and much more—all in a repeatable, automated way.
In this blog, we’ll walk through how to use Terraform to:
- Create a simple Lambda function
- Set up an API Gateway REST endpoint
- Integrate them together to handle HTTP requests
By the end, you’ll have a fully functional, serverless API deployed with just a few lines of code.
Prerequisites
Make sure you have the following:
- VS Code installed
- Terraform installed (
terraform -v
) - AWS CLI installed and configured (
aws configure
) - An AWS account
- Basic knowledge of terminal/command line
1. Project Setup in VS Code
1.1 Create Project Folder
api-gateway-lambda.
2. Write Lambda Code
In lambda/index.js
, paste this:
exports.handler = async (event) => {
return {
statusCode: 200,
body: JSON.stringify({ message: "Hello from Lambda!" }),
};
};
3. Zip the Lambda Function
From the terminal inside VS Code:
cd lambda
zip function.zip index.js
cd ..
4. Write Terraform Code.
main.tf
provider "aws" {
region = "us-east-1"
}
resource "aws_iam_role" "lambda_exec_role" {
name = "lambda_exec_role"
assume_role_policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Action = "sts:AssumeRole",
Effect = "Allow",
Principal = {
Service = "lambda.amazonaws.com"
}
}]
})
}
resource "aws_iam_role_policy_attachment" "lambda_policy" {
role = aws_iam_role.lambda_exec_role.name
policy_arn = "arn:aws:iam::aws:policy/service-role/AWSLambdaBasicExecutionRole"
}
resource "aws_lambda_function" "my_lambda" {
function_name = "my_lambda_function"
runtime = "nodejs18.x"
handler = "index.handler"
role = aws_iam_role.lambda_exec_role.arn
filename = "${path.module}/lambda/function.zip"
source_code_hash = filebase64sha256("${path.module}/lambda/function.zip")
}
resource "aws_apigatewayv2_api" "http_api" {
name = "http-api"
protocol_type = "HTTP"
}
resource "aws_apigatewayv2_integration" "lambda_integration" {
api_id = aws_apigatewayv2_api.http_api.id
integration_type = "AWS_PROXY"
integration_uri = aws_lambda_function.my_lambda.invoke_arn
integration_method = "POST"
payload_format_version = "2.0"
}
resource "aws_apigatewayv2_route" "lambda_route" {
api_id = aws_apigatewayv2_api.http_api.id
route_key = "GET /hello"
target = "integrations/${aws_apigatewayv2_integration.lambda_integration.id}"
}
resource "aws_apigatewayv2_stage" "default" {
api_id = aws_apigatewayv2_api.http_api.id
name = "$default"
auto_deploy = true
}
resource "aws_lambda_permission" "apigw_invoke" {
statement_id = "AllowAPIGatewayInvoke"
action = "lambda:InvokeFunction"
function_name = aws_lambda_function.my_lambda.function_name
principal = "apigateway.amazonaws.com"
source_arn = "${aws_apigatewayv2_api.http_api.execution_arn}//"
}
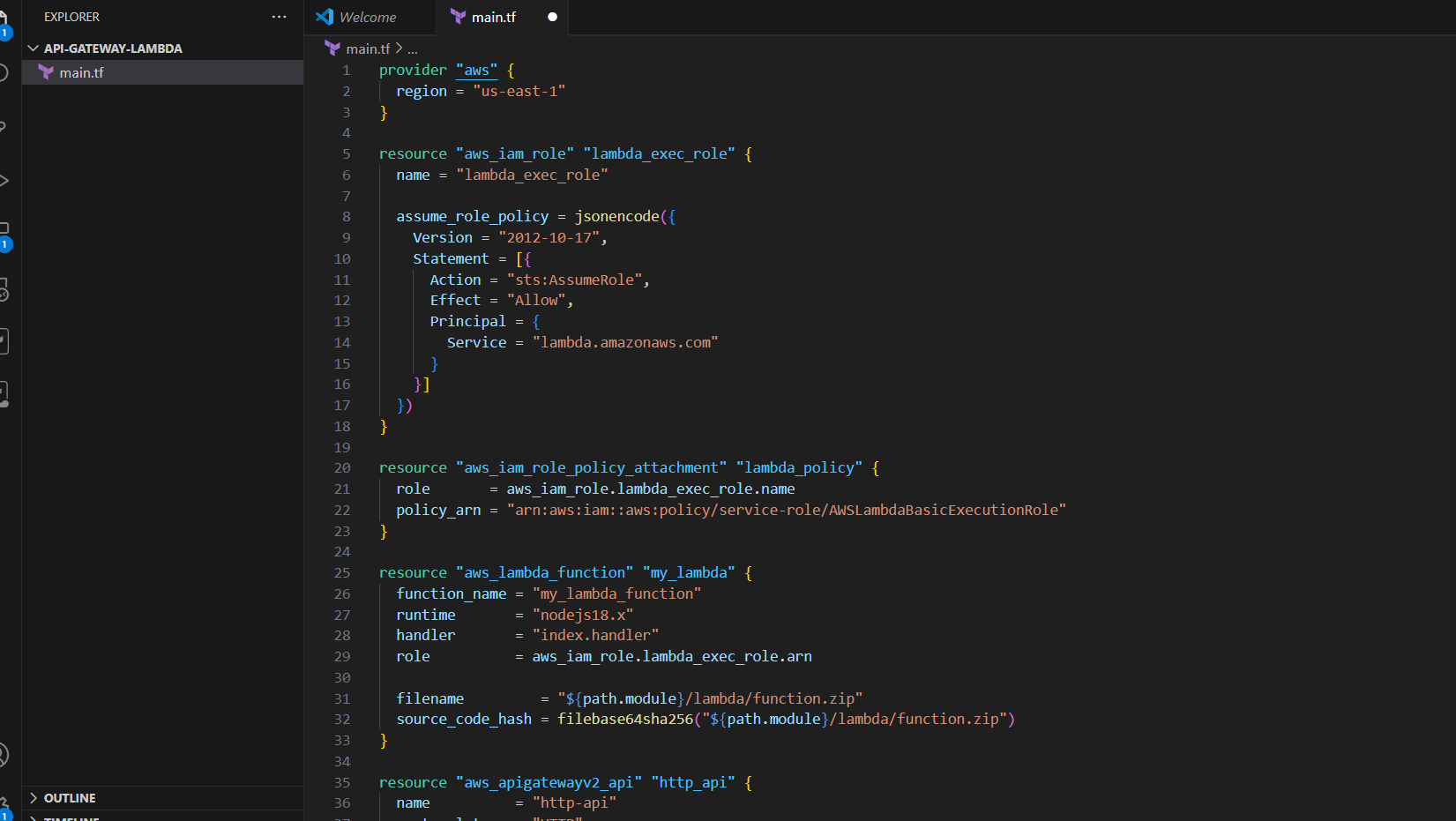
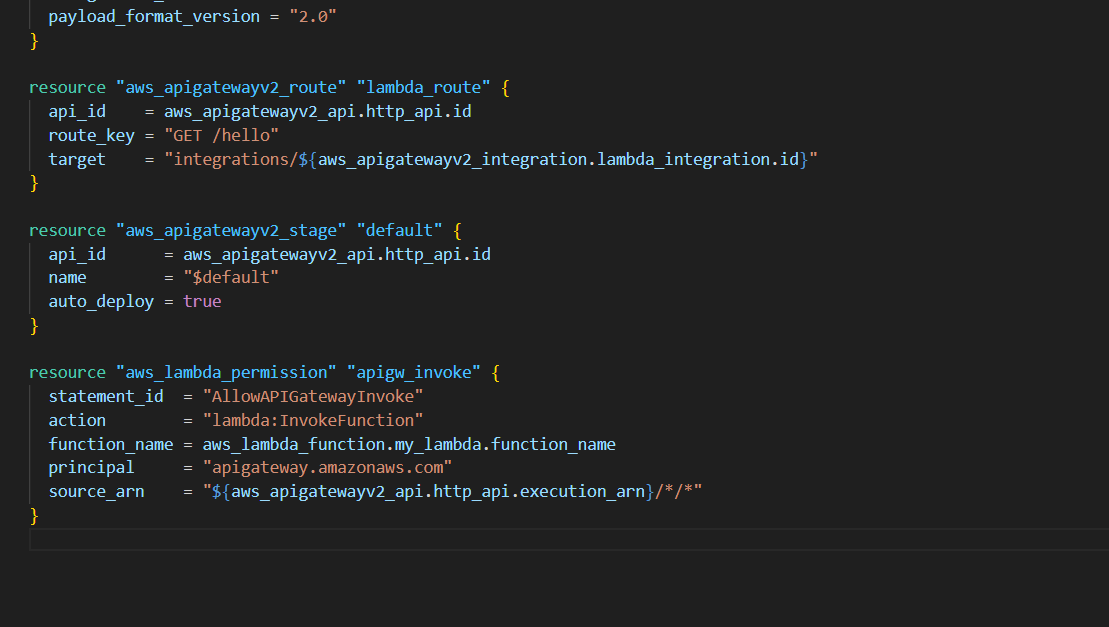
outputs.tf
output "api_endpoint" {
value = aws_apigatewayv2_api.http_api.api_endpoint
}
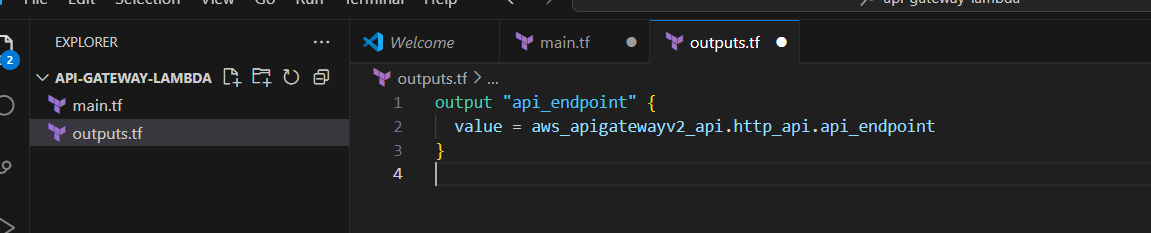
5. Deploy with Terraform
From the terminal in VS Code:
terraform init # Initialize Terraform
terraform plan # Optional: Review what will be created
terraform apply # Deploy everything (confirm with "yes")
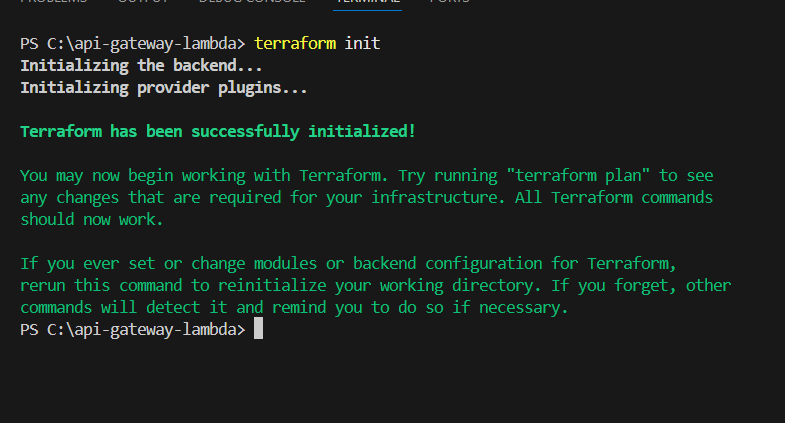
6. Clean Up.
terraform destroy
Conclusion.
In this post, we explored how to build a serverless API using AWS API Gateway, AWS Lambda, and Terraform. By defining our infrastructure as code, we achieved a setup that is not only repeatable and scalable but also easy to manage and version-controlled.
Using Terraform to provision resources like Lambda functions and API Gateway endpoints enables teams to move faster, reduce human error, and maintain consistent environments across development, staging, and production.
This simple use case—triggering a Lambda function via an API Gateway request—is just the beginning. With this foundation, you can extend your infrastructure to include custom domain names, request/response mapping, authentication, logging, monitoring, and much more.
As serverless architectures continue to grow in popularity, combining the power of AWS with the automation of Terraform is a smart and future-proof approach. Whether you’re building microservices, webhooks, or full APIs, you now have the tools to deploy them the right way.
Happy coding—and welcome to the world of serverless with Terraform!
Add a Comment